Display Firebase Data on HTML Page
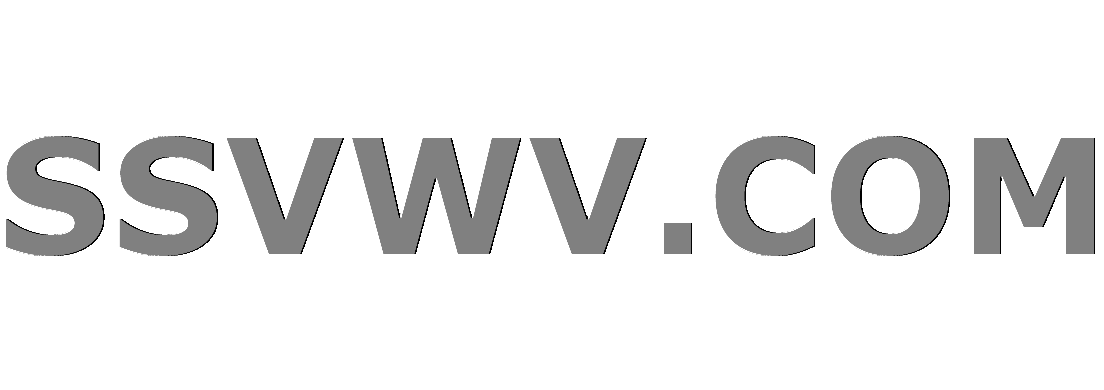
Multi tool use
up vote
0
down vote
favorite
I'm trying to display messages on a feed using the following template called feed.component.html
<div class="feed">
<div *ngFor="let message of feed | async" class="message">
<app-messages [chatMessage]="message"></app-messages>
</div>
</div>
I keep getting the following error, and am unable to display the messages I send to the Firebase Database on my html chat page.
ERROR Error: InvalidPipeArgument: '[object Object]' for pipe
'AsyncPipe'
I've also got my feed.component.ts
file as follows:
import { Component, OnInit, OnChanges } from '@angular/core';
import { ChatService } from '../services/chat.service';
import { Observable } from 'rxjs/observable';
import { ChatMessage } from '../models/chat-message.model';
import { AngularFireList } from 'angularfire2/database';
@Component({
selector: 'app-feed',
templateUrl: './feed.component.html',
styleUrls: ['./feed.component.css']
})
export class FeedComponent implements OnInit, OnChanges {
feed: AngularFireList<ChatMessage>;
constructor(private chat: ChatService) { }
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages();
}
ngOnChanges() {
this.feed = this.chat.getMessages();
}
}
I've also got my messages.components.ts
file below:
import { Component, OnInit, Input } from '@angular/core';
import { ChatService } from '../services/chat.service';
import { Observable } from 'rxjs/observable';
import { ChatMessage } from '../models/chat-message.model';
@Component({
selector: 'app-messages',
templateUrl: './messages.component.html',
styleUrls: ['./messages.component.css']
})
export class MessagesComponent implements OnInit {
@Input() chatMessage: ChatMessage;
userName: string;
userEmail: string;
messageContent: string;
timeStamp: Date = new Date();
constructor() { }
ngOnInit(chatMessage = this.chatMessage) {
this.messageContent = chatMessage.message;
this.timeStamp = chatMessage.timeSent;
this.userEmail = chatMessage.email;
this.userName = chatMessage.userName;
}
}
Can anyone please tell me what's wrong here and what I can do to fix it?
angular

add a comment |
up vote
0
down vote
favorite
I'm trying to display messages on a feed using the following template called feed.component.html
<div class="feed">
<div *ngFor="let message of feed | async" class="message">
<app-messages [chatMessage]="message"></app-messages>
</div>
</div>
I keep getting the following error, and am unable to display the messages I send to the Firebase Database on my html chat page.
ERROR Error: InvalidPipeArgument: '[object Object]' for pipe
'AsyncPipe'
I've also got my feed.component.ts
file as follows:
import { Component, OnInit, OnChanges } from '@angular/core';
import { ChatService } from '../services/chat.service';
import { Observable } from 'rxjs/observable';
import { ChatMessage } from '../models/chat-message.model';
import { AngularFireList } from 'angularfire2/database';
@Component({
selector: 'app-feed',
templateUrl: './feed.component.html',
styleUrls: ['./feed.component.css']
})
export class FeedComponent implements OnInit, OnChanges {
feed: AngularFireList<ChatMessage>;
constructor(private chat: ChatService) { }
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages();
}
ngOnChanges() {
this.feed = this.chat.getMessages();
}
}
I've also got my messages.components.ts
file below:
import { Component, OnInit, Input } from '@angular/core';
import { ChatService } from '../services/chat.service';
import { Observable } from 'rxjs/observable';
import { ChatMessage } from '../models/chat-message.model';
@Component({
selector: 'app-messages',
templateUrl: './messages.component.html',
styleUrls: ['./messages.component.css']
})
export class MessagesComponent implements OnInit {
@Input() chatMessage: ChatMessage;
userName: string;
userEmail: string;
messageContent: string;
timeStamp: Date = new Date();
constructor() { }
ngOnInit(chatMessage = this.chatMessage) {
this.messageContent = chatMessage.message;
this.timeStamp = chatMessage.timeSent;
this.userEmail = chatMessage.email;
this.userName = chatMessage.userName;
}
}
Can anyone please tell me what's wrong here and what I can do to fix it?
angular

Angularfire2 version? BTW, you should probably use anObservable
instead of anAngularFireList
. It's been removed, I think
– Edric
Mar 29 at 3:58
@Edric The AngularFire2 version I'm using is angularfire2: "^5.0.0-rc.6". By Observable, you mean FirebaseListObservable?
– jerome
Mar 29 at 4:01
No, just anObservable
onrxjs
– Edric
Mar 29 at 4:02
I'm really new to this, could you please show me how to do that? @Edric
– jerome
Mar 29 at 4:03
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to display messages on a feed using the following template called feed.component.html
<div class="feed">
<div *ngFor="let message of feed | async" class="message">
<app-messages [chatMessage]="message"></app-messages>
</div>
</div>
I keep getting the following error, and am unable to display the messages I send to the Firebase Database on my html chat page.
ERROR Error: InvalidPipeArgument: '[object Object]' for pipe
'AsyncPipe'
I've also got my feed.component.ts
file as follows:
import { Component, OnInit, OnChanges } from '@angular/core';
import { ChatService } from '../services/chat.service';
import { Observable } from 'rxjs/observable';
import { ChatMessage } from '../models/chat-message.model';
import { AngularFireList } from 'angularfire2/database';
@Component({
selector: 'app-feed',
templateUrl: './feed.component.html',
styleUrls: ['./feed.component.css']
})
export class FeedComponent implements OnInit, OnChanges {
feed: AngularFireList<ChatMessage>;
constructor(private chat: ChatService) { }
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages();
}
ngOnChanges() {
this.feed = this.chat.getMessages();
}
}
I've also got my messages.components.ts
file below:
import { Component, OnInit, Input } from '@angular/core';
import { ChatService } from '../services/chat.service';
import { Observable } from 'rxjs/observable';
import { ChatMessage } from '../models/chat-message.model';
@Component({
selector: 'app-messages',
templateUrl: './messages.component.html',
styleUrls: ['./messages.component.css']
})
export class MessagesComponent implements OnInit {
@Input() chatMessage: ChatMessage;
userName: string;
userEmail: string;
messageContent: string;
timeStamp: Date = new Date();
constructor() { }
ngOnInit(chatMessage = this.chatMessage) {
this.messageContent = chatMessage.message;
this.timeStamp = chatMessage.timeSent;
this.userEmail = chatMessage.email;
this.userName = chatMessage.userName;
}
}
Can anyone please tell me what's wrong here and what I can do to fix it?
angular

I'm trying to display messages on a feed using the following template called feed.component.html
<div class="feed">
<div *ngFor="let message of feed | async" class="message">
<app-messages [chatMessage]="message"></app-messages>
</div>
</div>
I keep getting the following error, and am unable to display the messages I send to the Firebase Database on my html chat page.
ERROR Error: InvalidPipeArgument: '[object Object]' for pipe
'AsyncPipe'
I've also got my feed.component.ts
file as follows:
import { Component, OnInit, OnChanges } from '@angular/core';
import { ChatService } from '../services/chat.service';
import { Observable } from 'rxjs/observable';
import { ChatMessage } from '../models/chat-message.model';
import { AngularFireList } from 'angularfire2/database';
@Component({
selector: 'app-feed',
templateUrl: './feed.component.html',
styleUrls: ['./feed.component.css']
})
export class FeedComponent implements OnInit, OnChanges {
feed: AngularFireList<ChatMessage>;
constructor(private chat: ChatService) { }
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages();
}
ngOnChanges() {
this.feed = this.chat.getMessages();
}
}
I've also got my messages.components.ts
file below:
import { Component, OnInit, Input } from '@angular/core';
import { ChatService } from '../services/chat.service';
import { Observable } from 'rxjs/observable';
import { ChatMessage } from '../models/chat-message.model';
@Component({
selector: 'app-messages',
templateUrl: './messages.component.html',
styleUrls: ['./messages.component.css']
})
export class MessagesComponent implements OnInit {
@Input() chatMessage: ChatMessage;
userName: string;
userEmail: string;
messageContent: string;
timeStamp: Date = new Date();
constructor() { }
ngOnInit(chatMessage = this.chatMessage) {
this.messageContent = chatMessage.message;
this.timeStamp = chatMessage.timeSent;
this.userEmail = chatMessage.email;
this.userName = chatMessage.userName;
}
}
Can anyone please tell me what's wrong here and what I can do to fix it?
angular

angular

edited Mar 29 at 10:23
Edric
6,12752542
6,12752542
asked Mar 29 at 3:57
jerome
6510
6510
Angularfire2 version? BTW, you should probably use anObservable
instead of anAngularFireList
. It's been removed, I think
– Edric
Mar 29 at 3:58
@Edric The AngularFire2 version I'm using is angularfire2: "^5.0.0-rc.6". By Observable, you mean FirebaseListObservable?
– jerome
Mar 29 at 4:01
No, just anObservable
onrxjs
– Edric
Mar 29 at 4:02
I'm really new to this, could you please show me how to do that? @Edric
– jerome
Mar 29 at 4:03
add a comment |
Angularfire2 version? BTW, you should probably use anObservable
instead of anAngularFireList
. It's been removed, I think
– Edric
Mar 29 at 3:58
@Edric The AngularFire2 version I'm using is angularfire2: "^5.0.0-rc.6". By Observable, you mean FirebaseListObservable?
– jerome
Mar 29 at 4:01
No, just anObservable
onrxjs
– Edric
Mar 29 at 4:02
I'm really new to this, could you please show me how to do that? @Edric
– jerome
Mar 29 at 4:03
Angularfire2 version? BTW, you should probably use an
Observable
instead of an AngularFireList
. It's been removed, I think– Edric
Mar 29 at 3:58
Angularfire2 version? BTW, you should probably use an
Observable
instead of an AngularFireList
. It's been removed, I think– Edric
Mar 29 at 3:58
@Edric The AngularFire2 version I'm using is angularfire2: "^5.0.0-rc.6". By Observable, you mean FirebaseListObservable?
– jerome
Mar 29 at 4:01
@Edric The AngularFire2 version I'm using is angularfire2: "^5.0.0-rc.6". By Observable, you mean FirebaseListObservable?
– jerome
Mar 29 at 4:01
No, just an
Observable
on rxjs
– Edric
Mar 29 at 4:02
No, just an
Observable
on rxjs
– Edric
Mar 29 at 4:02
I'm really new to this, could you please show me how to do that? @Edric
– jerome
Mar 29 at 4:03
I'm really new to this, could you please show me how to do that? @Edric
– jerome
Mar 29 at 4:03
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
What I did to solve the issue I was having was to change the following bit of code in the feed.component.ts file:
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages().valueChanges(); // Added `valueChanges`
}
I believe it then gets the object from the Firebase Database, and then *ngFor iterates through the object to give me the content
, userNames
and timestamps
for each message.
If there's something wrong with this answer, please let me know as I'm still quite new to this.
Cheers.
---UPDATE---
Another way I found was to use a service, where I used Subject as an observable to store my response. This made it accessible to all the components, which was easier than worrying about parent-child relationships.
Service File
const response = Subject();
const response$ = response.asObservable();
async getData() {
await someApiCall.subscribe((element) => {
this.response.next(element);
})
}
Component.ts
const data = ;
getDataFromService {
this.data = async() => this.service.getDatata();
}
View
<div *ngFor="let i of data>
<p>{{i | async}}</p>
</div>
ThevalueChanges
method is to basically anObservable
that emits with data whenever your database has been modified/added/updated/whatever you call it.
– Edric
Mar 29 at 10:20
By the way, you can also remove yourngOnChanges
method on your component file.
– Edric
Mar 29 at 10:23
add a comment |
up vote
0
down vote
AngularFireList
is to store the reference path, so that you can perform push()
, update()
and remove()
. You need to subscribe
to the list to get the values. For example
feedRef: AngularFireList<any>;
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feedRef = this.db.list('chats');
this.feeds = this.feedRef.valueChanges();
}
Beacuse you are getting reference from chat service you need to do
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feeds = this.chat.getMessages().valueChanges();
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
What I did to solve the issue I was having was to change the following bit of code in the feed.component.ts file:
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages().valueChanges(); // Added `valueChanges`
}
I believe it then gets the object from the Firebase Database, and then *ngFor iterates through the object to give me the content
, userNames
and timestamps
for each message.
If there's something wrong with this answer, please let me know as I'm still quite new to this.
Cheers.
---UPDATE---
Another way I found was to use a service, where I used Subject as an observable to store my response. This made it accessible to all the components, which was easier than worrying about parent-child relationships.
Service File
const response = Subject();
const response$ = response.asObservable();
async getData() {
await someApiCall.subscribe((element) => {
this.response.next(element);
})
}
Component.ts
const data = ;
getDataFromService {
this.data = async() => this.service.getDatata();
}
View
<div *ngFor="let i of data>
<p>{{i | async}}</p>
</div>
ThevalueChanges
method is to basically anObservable
that emits with data whenever your database has been modified/added/updated/whatever you call it.
– Edric
Mar 29 at 10:20
By the way, you can also remove yourngOnChanges
method on your component file.
– Edric
Mar 29 at 10:23
add a comment |
up vote
1
down vote
What I did to solve the issue I was having was to change the following bit of code in the feed.component.ts file:
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages().valueChanges(); // Added `valueChanges`
}
I believe it then gets the object from the Firebase Database, and then *ngFor iterates through the object to give me the content
, userNames
and timestamps
for each message.
If there's something wrong with this answer, please let me know as I'm still quite new to this.
Cheers.
---UPDATE---
Another way I found was to use a service, where I used Subject as an observable to store my response. This made it accessible to all the components, which was easier than worrying about parent-child relationships.
Service File
const response = Subject();
const response$ = response.asObservable();
async getData() {
await someApiCall.subscribe((element) => {
this.response.next(element);
})
}
Component.ts
const data = ;
getDataFromService {
this.data = async() => this.service.getDatata();
}
View
<div *ngFor="let i of data>
<p>{{i | async}}</p>
</div>
ThevalueChanges
method is to basically anObservable
that emits with data whenever your database has been modified/added/updated/whatever you call it.
– Edric
Mar 29 at 10:20
By the way, you can also remove yourngOnChanges
method on your component file.
– Edric
Mar 29 at 10:23
add a comment |
up vote
1
down vote
up vote
1
down vote
What I did to solve the issue I was having was to change the following bit of code in the feed.component.ts file:
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages().valueChanges(); // Added `valueChanges`
}
I believe it then gets the object from the Firebase Database, and then *ngFor iterates through the object to give me the content
, userNames
and timestamps
for each message.
If there's something wrong with this answer, please let me know as I'm still quite new to this.
Cheers.
---UPDATE---
Another way I found was to use a service, where I used Subject as an observable to store my response. This made it accessible to all the components, which was easier than worrying about parent-child relationships.
Service File
const response = Subject();
const response$ = response.asObservable();
async getData() {
await someApiCall.subscribe((element) => {
this.response.next(element);
})
}
Component.ts
const data = ;
getDataFromService {
this.data = async() => this.service.getDatata();
}
View
<div *ngFor="let i of data>
<p>{{i | async}}</p>
</div>
What I did to solve the issue I was having was to change the following bit of code in the feed.component.ts file:
ngOnInit() {
console.log("feed init...")
this.feed = this.chat.getMessages().valueChanges(); // Added `valueChanges`
}
I believe it then gets the object from the Firebase Database, and then *ngFor iterates through the object to give me the content
, userNames
and timestamps
for each message.
If there's something wrong with this answer, please let me know as I'm still quite new to this.
Cheers.
---UPDATE---
Another way I found was to use a service, where I used Subject as an observable to store my response. This made it accessible to all the components, which was easier than worrying about parent-child relationships.
Service File
const response = Subject();
const response$ = response.asObservable();
async getData() {
await someApiCall.subscribe((element) => {
this.response.next(element);
})
}
Component.ts
const data = ;
getDataFromService {
this.data = async() => this.service.getDatata();
}
View
<div *ngFor="let i of data>
<p>{{i | async}}</p>
</div>
edited Nov 11 at 13:49
answered Mar 29 at 4:59
jerome
6510
6510
ThevalueChanges
method is to basically anObservable
that emits with data whenever your database has been modified/added/updated/whatever you call it.
– Edric
Mar 29 at 10:20
By the way, you can also remove yourngOnChanges
method on your component file.
– Edric
Mar 29 at 10:23
add a comment |
ThevalueChanges
method is to basically anObservable
that emits with data whenever your database has been modified/added/updated/whatever you call it.
– Edric
Mar 29 at 10:20
By the way, you can also remove yourngOnChanges
method on your component file.
– Edric
Mar 29 at 10:23
The
valueChanges
method is to basically an Observable
that emits with data whenever your database has been modified/added/updated/whatever you call it.– Edric
Mar 29 at 10:20
The
valueChanges
method is to basically an Observable
that emits with data whenever your database has been modified/added/updated/whatever you call it.– Edric
Mar 29 at 10:20
By the way, you can also remove your
ngOnChanges
method on your component file.– Edric
Mar 29 at 10:23
By the way, you can also remove your
ngOnChanges
method on your component file.– Edric
Mar 29 at 10:23
add a comment |
up vote
0
down vote
AngularFireList
is to store the reference path, so that you can perform push()
, update()
and remove()
. You need to subscribe
to the list to get the values. For example
feedRef: AngularFireList<any>;
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feedRef = this.db.list('chats');
this.feeds = this.feedRef.valueChanges();
}
Beacuse you are getting reference from chat service you need to do
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feeds = this.chat.getMessages().valueChanges();
}
add a comment |
up vote
0
down vote
AngularFireList
is to store the reference path, so that you can perform push()
, update()
and remove()
. You need to subscribe
to the list to get the values. For example
feedRef: AngularFireList<any>;
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feedRef = this.db.list('chats');
this.feeds = this.feedRef.valueChanges();
}
Beacuse you are getting reference from chat service you need to do
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feeds = this.chat.getMessages().valueChanges();
}
add a comment |
up vote
0
down vote
up vote
0
down vote
AngularFireList
is to store the reference path, so that you can perform push()
, update()
and remove()
. You need to subscribe
to the list to get the values. For example
feedRef: AngularFireList<any>;
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feedRef = this.db.list('chats');
this.feeds = this.feedRef.valueChanges();
}
Beacuse you are getting reference from chat service you need to do
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feeds = this.chat.getMessages().valueChanges();
}
AngularFireList
is to store the reference path, so that you can perform push()
, update()
and remove()
. You need to subscribe
to the list to get the values. For example
feedRef: AngularFireList<any>;
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feedRef = this.db.list('chats');
this.feeds = this.feedRef.valueChanges();
}
Beacuse you are getting reference from chat service you need to do
feeds: Observable<ChatMessage>;
ngOnInit() {
this.feeds = this.chat.getMessages().valueChanges();
}
answered Mar 29 at 17:43
Hareesh
4,08532246
4,08532246
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49547793%2fdisplay-firebase-data-on-html-page%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HtpjKr qAxf tZO1A4P,PGAsqYIwbcs
Angularfire2 version? BTW, you should probably use an
Observable
instead of anAngularFireList
. It's been removed, I think– Edric
Mar 29 at 3:58
@Edric The AngularFire2 version I'm using is angularfire2: "^5.0.0-rc.6". By Observable, you mean FirebaseListObservable?
– jerome
Mar 29 at 4:01
No, just an
Observable
onrxjs
– Edric
Mar 29 at 4:02
I'm really new to this, could you please show me how to do that? @Edric
– jerome
Mar 29 at 4:03