Linking to images referenced in vuex store in Vue.js
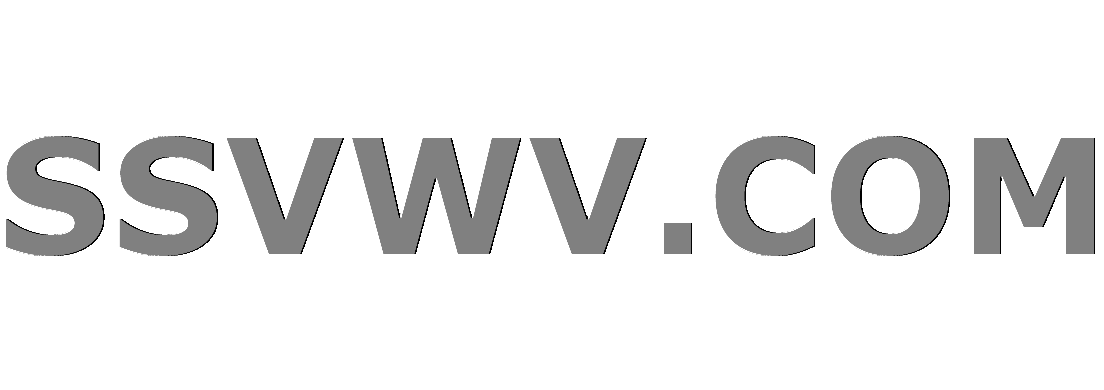
Multi tool use
I am using Vue.js for the first time so apologies if this is a basic question – I have set up the vue project with the vue-cli, vue-router and vuex if this information is helpful.
My main issue here is with displaying images or accessing assets. I am able to pull the appropriate data/state in from a data store via a 'getter' and iterate arrays, etc within it (for example, {{student.name}}
works perfectly) however when I attempt to display an image with <img :src='student.image'>
it fails to load and I get a broken link icon. I've done some research and it seems that there is a webpack naming convention for linking assets with ~/
or ~@/
however neither of these seem to work.
I've seen other examples where people simply link to a fixed asset from the component but because I am iterating the students
array I need a more programmatic method. I've seen some examples using computed()
properties but I feel like this should be unnecessary?
Below is the code from my component and the relevant parts of my store.js
file.
Store.js:
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
user: {
score: 0
},
students: [
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: '~/assets/images/students/advik-1.png'
},
{
id: 2,
name: 'Jake',
age: '19',
studying: 'Drama',
image: '~/assets/images/students/jake-1.png'
},
{
id: 3,
name: 'Mel',
age: '20',
studying: 'Civil Engineering',
image: '~/assets/images/students/mel-1.png'
},
{
id: 4,
name: 'Kaya',
age: '18',
studying: 'Law',
image: '~/assets/images/students/kaya-1.png'
}
]
},
mutations: {
},
methods: {
},
getters: {
getStudents: state => state.students
},
actions: {
}
})
Intros component:
<template>
<div>
<div class="m-background"></div>
<Brand />
<div class="l-container">
<div v-for="student in getStudents"
:key="student.id">
<img :src='student.image'>
<router-link class="m-btn m-btn--left m-btn__primary"
:to="{ name: 'home' }">{{ student.name }}
</router-link>
</div>
</div>
</div>
</template>
<script>
import { mapGetters } from 'vuex'
import Brand from '../../components/Brand'
export default {
components: {
Brand
},
computed: {
...mapGetters(['getStudents'])
},
name: 'Intros'
}
</script>
<style>
</style>
Thank you so much!
javascript vue.js url-routing vuex
add a comment |
I am using Vue.js for the first time so apologies if this is a basic question – I have set up the vue project with the vue-cli, vue-router and vuex if this information is helpful.
My main issue here is with displaying images or accessing assets. I am able to pull the appropriate data/state in from a data store via a 'getter' and iterate arrays, etc within it (for example, {{student.name}}
works perfectly) however when I attempt to display an image with <img :src='student.image'>
it fails to load and I get a broken link icon. I've done some research and it seems that there is a webpack naming convention for linking assets with ~/
or ~@/
however neither of these seem to work.
I've seen other examples where people simply link to a fixed asset from the component but because I am iterating the students
array I need a more programmatic method. I've seen some examples using computed()
properties but I feel like this should be unnecessary?
Below is the code from my component and the relevant parts of my store.js
file.
Store.js:
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
user: {
score: 0
},
students: [
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: '~/assets/images/students/advik-1.png'
},
{
id: 2,
name: 'Jake',
age: '19',
studying: 'Drama',
image: '~/assets/images/students/jake-1.png'
},
{
id: 3,
name: 'Mel',
age: '20',
studying: 'Civil Engineering',
image: '~/assets/images/students/mel-1.png'
},
{
id: 4,
name: 'Kaya',
age: '18',
studying: 'Law',
image: '~/assets/images/students/kaya-1.png'
}
]
},
mutations: {
},
methods: {
},
getters: {
getStudents: state => state.students
},
actions: {
}
})
Intros component:
<template>
<div>
<div class="m-background"></div>
<Brand />
<div class="l-container">
<div v-for="student in getStudents"
:key="student.id">
<img :src='student.image'>
<router-link class="m-btn m-btn--left m-btn__primary"
:to="{ name: 'home' }">{{ student.name }}
</router-link>
</div>
</div>
</div>
</template>
<script>
import { mapGetters } from 'vuex'
import Brand from '../../components/Brand'
export default {
components: {
Brand
},
computed: {
...mapGetters(['getStudents'])
},
name: 'Intros'
}
</script>
<style>
</style>
Thank you so much!
javascript vue.js url-routing vuex
add a comment |
I am using Vue.js for the first time so apologies if this is a basic question – I have set up the vue project with the vue-cli, vue-router and vuex if this information is helpful.
My main issue here is with displaying images or accessing assets. I am able to pull the appropriate data/state in from a data store via a 'getter' and iterate arrays, etc within it (for example, {{student.name}}
works perfectly) however when I attempt to display an image with <img :src='student.image'>
it fails to load and I get a broken link icon. I've done some research and it seems that there is a webpack naming convention for linking assets with ~/
or ~@/
however neither of these seem to work.
I've seen other examples where people simply link to a fixed asset from the component but because I am iterating the students
array I need a more programmatic method. I've seen some examples using computed()
properties but I feel like this should be unnecessary?
Below is the code from my component and the relevant parts of my store.js
file.
Store.js:
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
user: {
score: 0
},
students: [
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: '~/assets/images/students/advik-1.png'
},
{
id: 2,
name: 'Jake',
age: '19',
studying: 'Drama',
image: '~/assets/images/students/jake-1.png'
},
{
id: 3,
name: 'Mel',
age: '20',
studying: 'Civil Engineering',
image: '~/assets/images/students/mel-1.png'
},
{
id: 4,
name: 'Kaya',
age: '18',
studying: 'Law',
image: '~/assets/images/students/kaya-1.png'
}
]
},
mutations: {
},
methods: {
},
getters: {
getStudents: state => state.students
},
actions: {
}
})
Intros component:
<template>
<div>
<div class="m-background"></div>
<Brand />
<div class="l-container">
<div v-for="student in getStudents"
:key="student.id">
<img :src='student.image'>
<router-link class="m-btn m-btn--left m-btn__primary"
:to="{ name: 'home' }">{{ student.name }}
</router-link>
</div>
</div>
</div>
</template>
<script>
import { mapGetters } from 'vuex'
import Brand from '../../components/Brand'
export default {
components: {
Brand
},
computed: {
...mapGetters(['getStudents'])
},
name: 'Intros'
}
</script>
<style>
</style>
Thank you so much!
javascript vue.js url-routing vuex
I am using Vue.js for the first time so apologies if this is a basic question – I have set up the vue project with the vue-cli, vue-router and vuex if this information is helpful.
My main issue here is with displaying images or accessing assets. I am able to pull the appropriate data/state in from a data store via a 'getter' and iterate arrays, etc within it (for example, {{student.name}}
works perfectly) however when I attempt to display an image with <img :src='student.image'>
it fails to load and I get a broken link icon. I've done some research and it seems that there is a webpack naming convention for linking assets with ~/
or ~@/
however neither of these seem to work.
I've seen other examples where people simply link to a fixed asset from the component but because I am iterating the students
array I need a more programmatic method. I've seen some examples using computed()
properties but I feel like this should be unnecessary?
Below is the code from my component and the relevant parts of my store.js
file.
Store.js:
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
user: {
score: 0
},
students: [
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: '~/assets/images/students/advik-1.png'
},
{
id: 2,
name: 'Jake',
age: '19',
studying: 'Drama',
image: '~/assets/images/students/jake-1.png'
},
{
id: 3,
name: 'Mel',
age: '20',
studying: 'Civil Engineering',
image: '~/assets/images/students/mel-1.png'
},
{
id: 4,
name: 'Kaya',
age: '18',
studying: 'Law',
image: '~/assets/images/students/kaya-1.png'
}
]
},
mutations: {
},
methods: {
},
getters: {
getStudents: state => state.students
},
actions: {
}
})
Intros component:
<template>
<div>
<div class="m-background"></div>
<Brand />
<div class="l-container">
<div v-for="student in getStudents"
:key="student.id">
<img :src='student.image'>
<router-link class="m-btn m-btn--left m-btn__primary"
:to="{ name: 'home' }">{{ student.name }}
</router-link>
</div>
</div>
</div>
</template>
<script>
import { mapGetters } from 'vuex'
import Brand from '../../components/Brand'
export default {
components: {
Brand
},
computed: {
...mapGetters(['getStudents'])
},
name: 'Intros'
}
</script>
<style>
</style>
Thank you so much!
javascript vue.js url-routing vuex
javascript vue.js url-routing vuex
edited Nov 21 '18 at 12:56
Jack Clarke
asked Nov 21 '18 at 12:31


Jack ClarkeJack Clarke
809
809
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
:src='student.image'
(v-binding) is executed at runtime, but webpack aliases work in compile time. So you have to wrap the aliased file path in require
.
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: require('~@/assets/images/students/advik-1.png')
}
Thank you so much @Jns! Do you also happen to know how I would link to a directory one folder up the file tree? Mystore.js
file is in a 'store' folder at the same level as 'assets'? I've tried variations on../
such asrequire('~@../assets/images/students/advik-1.png')
but it doesn't seem to work?
– Jack Clarke
Nov 21 '18 at 13:17
I feel like there must be something else I am doing wrong @Jns, now I get a failed to compile error which is good, but it says:Module not found: Error: Can't resolve '~@/store/advik-1.png' in /my-project/src/store' @ ./src/store/store.js 18:13-44 @ ./src/main.js @ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./src/main.js
– Jack Clarke
Nov 21 '18 at 13:31
Could you create a small fiddle at codesandbox.io, which has a simliar structure as your project, please?
– Jns
Nov 21 '18 at 13:34
2
If your file is just in the top-levelassets
folder, you don't want the~
-- just userequire("@/assets/.../whatever.png")
– Daniel Beck
Nov 21 '18 at 13:34
Hi both @DanielBeck and @Jns – thanks for your help – my assets folder was in the top level so the straightforward@
has worked a treat, thank you! I wonder if either of you know of a website that has documentation on this? I can't find a reason why this naming convention is the case? Why the~
and/or the@
?
– Jack Clarke
Nov 21 '18 at 13:55
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53412106%2flinking-to-images-referenced-in-vuex-store-in-vue-js%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
:src='student.image'
(v-binding) is executed at runtime, but webpack aliases work in compile time. So you have to wrap the aliased file path in require
.
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: require('~@/assets/images/students/advik-1.png')
}
Thank you so much @Jns! Do you also happen to know how I would link to a directory one folder up the file tree? Mystore.js
file is in a 'store' folder at the same level as 'assets'? I've tried variations on../
such asrequire('~@../assets/images/students/advik-1.png')
but it doesn't seem to work?
– Jack Clarke
Nov 21 '18 at 13:17
I feel like there must be something else I am doing wrong @Jns, now I get a failed to compile error which is good, but it says:Module not found: Error: Can't resolve '~@/store/advik-1.png' in /my-project/src/store' @ ./src/store/store.js 18:13-44 @ ./src/main.js @ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./src/main.js
– Jack Clarke
Nov 21 '18 at 13:31
Could you create a small fiddle at codesandbox.io, which has a simliar structure as your project, please?
– Jns
Nov 21 '18 at 13:34
2
If your file is just in the top-levelassets
folder, you don't want the~
-- just userequire("@/assets/.../whatever.png")
– Daniel Beck
Nov 21 '18 at 13:34
Hi both @DanielBeck and @Jns – thanks for your help – my assets folder was in the top level so the straightforward@
has worked a treat, thank you! I wonder if either of you know of a website that has documentation on this? I can't find a reason why this naming convention is the case? Why the~
and/or the@
?
– Jack Clarke
Nov 21 '18 at 13:55
|
show 1 more comment
:src='student.image'
(v-binding) is executed at runtime, but webpack aliases work in compile time. So you have to wrap the aliased file path in require
.
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: require('~@/assets/images/students/advik-1.png')
}
Thank you so much @Jns! Do you also happen to know how I would link to a directory one folder up the file tree? Mystore.js
file is in a 'store' folder at the same level as 'assets'? I've tried variations on../
such asrequire('~@../assets/images/students/advik-1.png')
but it doesn't seem to work?
– Jack Clarke
Nov 21 '18 at 13:17
I feel like there must be something else I am doing wrong @Jns, now I get a failed to compile error which is good, but it says:Module not found: Error: Can't resolve '~@/store/advik-1.png' in /my-project/src/store' @ ./src/store/store.js 18:13-44 @ ./src/main.js @ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./src/main.js
– Jack Clarke
Nov 21 '18 at 13:31
Could you create a small fiddle at codesandbox.io, which has a simliar structure as your project, please?
– Jns
Nov 21 '18 at 13:34
2
If your file is just in the top-levelassets
folder, you don't want the~
-- just userequire("@/assets/.../whatever.png")
– Daniel Beck
Nov 21 '18 at 13:34
Hi both @DanielBeck and @Jns – thanks for your help – my assets folder was in the top level so the straightforward@
has worked a treat, thank you! I wonder if either of you know of a website that has documentation on this? I can't find a reason why this naming convention is the case? Why the~
and/or the@
?
– Jack Clarke
Nov 21 '18 at 13:55
|
show 1 more comment
:src='student.image'
(v-binding) is executed at runtime, but webpack aliases work in compile time. So you have to wrap the aliased file path in require
.
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: require('~@/assets/images/students/advik-1.png')
}
:src='student.image'
(v-binding) is executed at runtime, but webpack aliases work in compile time. So you have to wrap the aliased file path in require
.
{
id: 1,
name: 'Advik',
age: '19',
studying: 'Physiotherapy',
image: require('~@/assets/images/students/advik-1.png')
}
answered Nov 21 '18 at 13:11


JnsJns
1,0431513
1,0431513
Thank you so much @Jns! Do you also happen to know how I would link to a directory one folder up the file tree? Mystore.js
file is in a 'store' folder at the same level as 'assets'? I've tried variations on../
such asrequire('~@../assets/images/students/advik-1.png')
but it doesn't seem to work?
– Jack Clarke
Nov 21 '18 at 13:17
I feel like there must be something else I am doing wrong @Jns, now I get a failed to compile error which is good, but it says:Module not found: Error: Can't resolve '~@/store/advik-1.png' in /my-project/src/store' @ ./src/store/store.js 18:13-44 @ ./src/main.js @ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./src/main.js
– Jack Clarke
Nov 21 '18 at 13:31
Could you create a small fiddle at codesandbox.io, which has a simliar structure as your project, please?
– Jns
Nov 21 '18 at 13:34
2
If your file is just in the top-levelassets
folder, you don't want the~
-- just userequire("@/assets/.../whatever.png")
– Daniel Beck
Nov 21 '18 at 13:34
Hi both @DanielBeck and @Jns – thanks for your help – my assets folder was in the top level so the straightforward@
has worked a treat, thank you! I wonder if either of you know of a website that has documentation on this? I can't find a reason why this naming convention is the case? Why the~
and/or the@
?
– Jack Clarke
Nov 21 '18 at 13:55
|
show 1 more comment
Thank you so much @Jns! Do you also happen to know how I would link to a directory one folder up the file tree? Mystore.js
file is in a 'store' folder at the same level as 'assets'? I've tried variations on../
such asrequire('~@../assets/images/students/advik-1.png')
but it doesn't seem to work?
– Jack Clarke
Nov 21 '18 at 13:17
I feel like there must be something else I am doing wrong @Jns, now I get a failed to compile error which is good, but it says:Module not found: Error: Can't resolve '~@/store/advik-1.png' in /my-project/src/store' @ ./src/store/store.js 18:13-44 @ ./src/main.js @ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./src/main.js
– Jack Clarke
Nov 21 '18 at 13:31
Could you create a small fiddle at codesandbox.io, which has a simliar structure as your project, please?
– Jns
Nov 21 '18 at 13:34
2
If your file is just in the top-levelassets
folder, you don't want the~
-- just userequire("@/assets/.../whatever.png")
– Daniel Beck
Nov 21 '18 at 13:34
Hi both @DanielBeck and @Jns – thanks for your help – my assets folder was in the top level so the straightforward@
has worked a treat, thank you! I wonder if either of you know of a website that has documentation on this? I can't find a reason why this naming convention is the case? Why the~
and/or the@
?
– Jack Clarke
Nov 21 '18 at 13:55
Thank you so much @Jns! Do you also happen to know how I would link to a directory one folder up the file tree? My
store.js
file is in a 'store' folder at the same level as 'assets'? I've tried variations on ../
such as require('~@../assets/images/students/advik-1.png')
but it doesn't seem to work?– Jack Clarke
Nov 21 '18 at 13:17
Thank you so much @Jns! Do you also happen to know how I would link to a directory one folder up the file tree? My
store.js
file is in a 'store' folder at the same level as 'assets'? I've tried variations on ../
such as require('~@../assets/images/students/advik-1.png')
but it doesn't seem to work?– Jack Clarke
Nov 21 '18 at 13:17
I feel like there must be something else I am doing wrong @Jns, now I get a failed to compile error which is good, but it says:
Module not found: Error: Can't resolve '~@/store/advik-1.png' in /my-project/src/store' @ ./src/store/store.js 18:13-44 @ ./src/main.js @ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./src/main.js
– Jack Clarke
Nov 21 '18 at 13:31
I feel like there must be something else I am doing wrong @Jns, now I get a failed to compile error which is good, but it says:
Module not found: Error: Can't resolve '~@/store/advik-1.png' in /my-project/src/store' @ ./src/store/store.js 18:13-44 @ ./src/main.js @ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./src/main.js
– Jack Clarke
Nov 21 '18 at 13:31
Could you create a small fiddle at codesandbox.io, which has a simliar structure as your project, please?
– Jns
Nov 21 '18 at 13:34
Could you create a small fiddle at codesandbox.io, which has a simliar structure as your project, please?
– Jns
Nov 21 '18 at 13:34
2
2
If your file is just in the top-level
assets
folder, you don't want the ~
-- just use require("@/assets/.../whatever.png")
– Daniel Beck
Nov 21 '18 at 13:34
If your file is just in the top-level
assets
folder, you don't want the ~
-- just use require("@/assets/.../whatever.png")
– Daniel Beck
Nov 21 '18 at 13:34
Hi both @DanielBeck and @Jns – thanks for your help – my assets folder was in the top level so the straightforward
@
has worked a treat, thank you! I wonder if either of you know of a website that has documentation on this? I can't find a reason why this naming convention is the case? Why the ~
and/or the @
?– Jack Clarke
Nov 21 '18 at 13:55
Hi both @DanielBeck and @Jns – thanks for your help – my assets folder was in the top level so the straightforward
@
has worked a treat, thank you! I wonder if either of you know of a website that has documentation on this? I can't find a reason why this naming convention is the case? Why the ~
and/or the @
?– Jack Clarke
Nov 21 '18 at 13:55
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53412106%2flinking-to-images-referenced-in-vuex-store-in-vue-js%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S2TyC04paP