Can't set state when onRadiusChanged
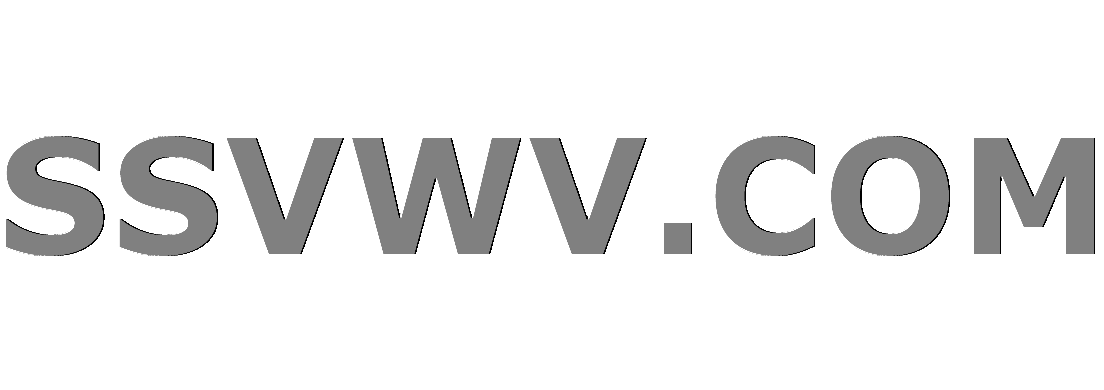
Multi tool use
up vote
0
down vote
favorite
I can not set state in this code. I understand it because I did not bind the function getvalue. Otherwise I cannot call this.radius. I this code this.radius is 5000. If I changed it to
getvalues = (e)=> { console.log(e)}
it returns undefined.
So what do I need to do
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues(e) {
this.setState({'radius': this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
node.js reactjs

add a comment |
up vote
0
down vote
favorite
I can not set state in this code. I understand it because I did not bind the function getvalue. Otherwise I cannot call this.radius. I this code this.radius is 5000. If I changed it to
getvalues = (e)=> { console.log(e)}
it returns undefined.
So what do I need to do
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues(e) {
this.setState({'radius': this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
node.js reactjs

add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I can not set state in this code. I understand it because I did not bind the function getvalue. Otherwise I cannot call this.radius. I this code this.radius is 5000. If I changed it to
getvalues = (e)=> { console.log(e)}
it returns undefined.
So what do I need to do
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues(e) {
this.setState({'radius': this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
node.js reactjs

I can not set state in this code. I understand it because I did not bind the function getvalue. Otherwise I cannot call this.radius. I this code this.radius is 5000. If I changed it to
getvalues = (e)=> { console.log(e)}
it returns undefined.
So what do I need to do
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues(e) {
this.setState({'radius': this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
node.js reactjs

node.js reactjs

edited Nov 11 at 15:49


Thakur Karthik
389213
389213
asked Nov 11 at 11:16
Wouter
11219
11219
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
I found the answer. You need to ref the circle component and then you can call the getValues function. Also you need tot return false the shouldComponentUpdate
This is the code
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000
};
}
mapMounted = ref => {
this.map = ref;
};
shouldComponentUpdate(nextProps, nextState) {
return false;
}
getvalues = e => {
const radius = this.map.getRadius();
const center = this.map.getCenter();
this.setState({ radius: radius });
this.setState({
"location.lat": center.lat(),
"location.lng": center.lng()
});
};
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap defaultZoom={11} defaultCenter={this.state.location}>
<Circle
ref={this.mapMounted.bind(this)}
radius={this.state.radius}
editable={true}
onRadiusChanged={props.getval}
onDragEnd={props.getval}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
getval={this.getvalues.bind(this)}
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
add a comment |
up vote
-1
down vote
Please try this, the issue seems to be with binding.
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues = (e) => {
this.setState({radius: this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I found the answer. You need to ref the circle component and then you can call the getValues function. Also you need tot return false the shouldComponentUpdate
This is the code
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000
};
}
mapMounted = ref => {
this.map = ref;
};
shouldComponentUpdate(nextProps, nextState) {
return false;
}
getvalues = e => {
const radius = this.map.getRadius();
const center = this.map.getCenter();
this.setState({ radius: radius });
this.setState({
"location.lat": center.lat(),
"location.lng": center.lng()
});
};
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap defaultZoom={11} defaultCenter={this.state.location}>
<Circle
ref={this.mapMounted.bind(this)}
radius={this.state.radius}
editable={true}
onRadiusChanged={props.getval}
onDragEnd={props.getval}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
getval={this.getvalues.bind(this)}
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
add a comment |
up vote
0
down vote
I found the answer. You need to ref the circle component and then you can call the getValues function. Also you need tot return false the shouldComponentUpdate
This is the code
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000
};
}
mapMounted = ref => {
this.map = ref;
};
shouldComponentUpdate(nextProps, nextState) {
return false;
}
getvalues = e => {
const radius = this.map.getRadius();
const center = this.map.getCenter();
this.setState({ radius: radius });
this.setState({
"location.lat": center.lat(),
"location.lng": center.lng()
});
};
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap defaultZoom={11} defaultCenter={this.state.location}>
<Circle
ref={this.mapMounted.bind(this)}
radius={this.state.radius}
editable={true}
onRadiusChanged={props.getval}
onDragEnd={props.getval}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
getval={this.getvalues.bind(this)}
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
add a comment |
up vote
0
down vote
up vote
0
down vote
I found the answer. You need to ref the circle component and then you can call the getValues function. Also you need tot return false the shouldComponentUpdate
This is the code
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000
};
}
mapMounted = ref => {
this.map = ref;
};
shouldComponentUpdate(nextProps, nextState) {
return false;
}
getvalues = e => {
const radius = this.map.getRadius();
const center = this.map.getCenter();
this.setState({ radius: radius });
this.setState({
"location.lat": center.lat(),
"location.lng": center.lng()
});
};
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap defaultZoom={11} defaultCenter={this.state.location}>
<Circle
ref={this.mapMounted.bind(this)}
radius={this.state.radius}
editable={true}
onRadiusChanged={props.getval}
onDragEnd={props.getval}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
getval={this.getvalues.bind(this)}
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
I found the answer. You need to ref the circle component and then you can call the getValues function. Also you need tot return false the shouldComponentUpdate
This is the code
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000
};
}
mapMounted = ref => {
this.map = ref;
};
shouldComponentUpdate(nextProps, nextState) {
return false;
}
getvalues = e => {
const radius = this.map.getRadius();
const center = this.map.getCenter();
this.setState({ radius: radius });
this.setState({
"location.lat": center.lat(),
"location.lng": center.lng()
});
};
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap defaultZoom={11} defaultCenter={this.state.location}>
<Circle
ref={this.mapMounted.bind(this)}
radius={this.state.radius}
editable={true}
onRadiusChanged={props.getval}
onDragEnd={props.getval}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
getval={this.getvalues.bind(this)}
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
answered Nov 11 at 15:50
Wouter
11219
11219
add a comment |
add a comment |
up vote
-1
down vote
Please try this, the issue seems to be with binding.
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues = (e) => {
this.setState({radius: this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
add a comment |
up vote
-1
down vote
Please try this, the issue seems to be with binding.
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues = (e) => {
this.setState({radius: this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
add a comment |
up vote
-1
down vote
up vote
-1
down vote
Please try this, the issue seems to be with binding.
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues = (e) => {
this.setState({radius: this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
Please try this, the issue seems to be with binding.
class Mapspage extends React.Component {
constructor(props) {
super(props);
this.state = {
location: { lat: 52.2387683, lng: 5.8322737 },
position: ,
radius: 5000,
};
}
getvalues = (e) => {
this.setState({radius: this.radius});
}
render() {
const MapWithAMarker = withGoogleMap(props => (
<GoogleMap
defaultZoom={11}
defaultCenter={this.state.location}
>
<Circle
radius={this.state.radius}
editable={true}
onRadiusChanged={this.getvalues}
onDragEnd={this.getvalues}
draggable={true}
visible={true}
center={this.state.location}
/>
</GoogleMap>
));
return (
<div className="box box-default">
<MapWithAMarker
containerElement={<div style={{ height: `400px` }} />}
mapElement={<div style={{ height: `100%` }} />}
/>
</div>
);
}
}
answered Nov 11 at 15:54


Muneer PP
694
694
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53248165%2fcant-set-state-when-onradiuschanged%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qjtT2,GCJQ TtStk,t68zxSKWg0IgFTOJBxW5HjsSE U5JTa Rse,TXEOxa XsRvl MxPnuNChn6PC1l4VHDn9zJJX D1HE2j4hRu,618K5