Spring webflux doesn't populate custom object from path variables with @ModelAttribute
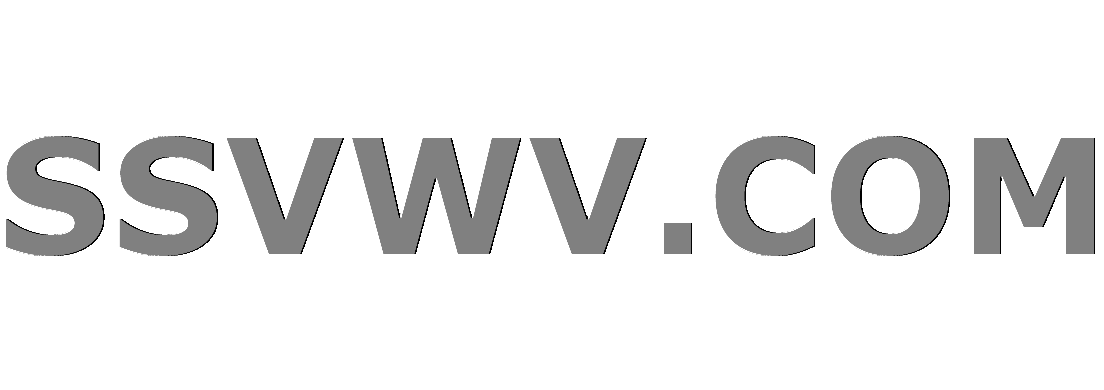
Multi tool use
up vote
1
down vote
favorite
I'm trying to adapt an application that works well with Spring MVC but that has a different behavior with Spring WebFlux
Here's my code with Spring Boot 5 - Spring MVC :
The controller :
@RestController
public class MyRestController {
@GetMapping("/test/{id}/{label}")
public ResponseEntity<Payload> test(@ModelAttribute Payload payload) {
return new ResponseEntity<>(payload,HttpStatus.OK);
}
}
The Payload object :
public class Payload {
@NotNull
private int id;
private String label;
public Payload() {}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
My pom.xml
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
I don't have written any custom converter, Spring populate my payload object automatically, everything is fine.
When I call :
http://localhost:8080/test/25/helloWorld
Response is
{"id":25,"label":"helloWorld"}
Then, I only change my pom.xml, switching from web to webflux :
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
</dependencies>
And my Payload Object isn't populated anymore.
When I call :
http://localhost:8080/test/25/helloWorld
Response is
{"id":0,"label":null}
I know I could write a converter and register it with @ControllerAdvice, but I can't imagine that there isn't an automated solution to make it works again as it has always worked with Spring Web.
Does anyone already encountered the same problem as me ?
Thanks,
Julien
java spring spring-mvc spring-boot spring-webflux
add a comment |
up vote
1
down vote
favorite
I'm trying to adapt an application that works well with Spring MVC but that has a different behavior with Spring WebFlux
Here's my code with Spring Boot 5 - Spring MVC :
The controller :
@RestController
public class MyRestController {
@GetMapping("/test/{id}/{label}")
public ResponseEntity<Payload> test(@ModelAttribute Payload payload) {
return new ResponseEntity<>(payload,HttpStatus.OK);
}
}
The Payload object :
public class Payload {
@NotNull
private int id;
private String label;
public Payload() {}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
My pom.xml
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
I don't have written any custom converter, Spring populate my payload object automatically, everything is fine.
When I call :
http://localhost:8080/test/25/helloWorld
Response is
{"id":25,"label":"helloWorld"}
Then, I only change my pom.xml, switching from web to webflux :
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
</dependencies>
And my Payload Object isn't populated anymore.
When I call :
http://localhost:8080/test/25/helloWorld
Response is
{"id":0,"label":null}
I know I could write a converter and register it with @ControllerAdvice, but I can't imagine that there isn't an automated solution to make it works again as it has always worked with Spring Web.
Does anyone already encountered the same problem as me ?
Thanks,
Julien
java spring spring-mvc spring-boot spring-webflux
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to adapt an application that works well with Spring MVC but that has a different behavior with Spring WebFlux
Here's my code with Spring Boot 5 - Spring MVC :
The controller :
@RestController
public class MyRestController {
@GetMapping("/test/{id}/{label}")
public ResponseEntity<Payload> test(@ModelAttribute Payload payload) {
return new ResponseEntity<>(payload,HttpStatus.OK);
}
}
The Payload object :
public class Payload {
@NotNull
private int id;
private String label;
public Payload() {}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
My pom.xml
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
I don't have written any custom converter, Spring populate my payload object automatically, everything is fine.
When I call :
http://localhost:8080/test/25/helloWorld
Response is
{"id":25,"label":"helloWorld"}
Then, I only change my pom.xml, switching from web to webflux :
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
</dependencies>
And my Payload Object isn't populated anymore.
When I call :
http://localhost:8080/test/25/helloWorld
Response is
{"id":0,"label":null}
I know I could write a converter and register it with @ControllerAdvice, but I can't imagine that there isn't an automated solution to make it works again as it has always worked with Spring Web.
Does anyone already encountered the same problem as me ?
Thanks,
Julien
java spring spring-mvc spring-boot spring-webflux
I'm trying to adapt an application that works well with Spring MVC but that has a different behavior with Spring WebFlux
Here's my code with Spring Boot 5 - Spring MVC :
The controller :
@RestController
public class MyRestController {
@GetMapping("/test/{id}/{label}")
public ResponseEntity<Payload> test(@ModelAttribute Payload payload) {
return new ResponseEntity<>(payload,HttpStatus.OK);
}
}
The Payload object :
public class Payload {
@NotNull
private int id;
private String label;
public Payload() {}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
My pom.xml
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
I don't have written any custom converter, Spring populate my payload object automatically, everything is fine.
When I call :
http://localhost:8080/test/25/helloWorld
Response is
{"id":25,"label":"helloWorld"}
Then, I only change my pom.xml, switching from web to webflux :
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
</dependencies>
And my Payload Object isn't populated anymore.
When I call :
http://localhost:8080/test/25/helloWorld
Response is
{"id":0,"label":null}
I know I could write a converter and register it with @ControllerAdvice, but I can't imagine that there isn't an automated solution to make it works again as it has always worked with Spring Web.
Does anyone already encountered the same problem as me ?
Thanks,
Julien
java spring spring-mvc spring-boot spring-webflux
java spring spring-mvc spring-boot spring-webflux
edited Nov 11 at 22:43


chrylis
50k1678115
50k1678115
asked Nov 11 at 22:38
Julien O
7616
7616
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
The Spring WebFlux reference documentation about @ModelAttribute
does not mention URI path variables, unlike the same section in the reference documentation for Spring MVC:
The Pet instance above is resolved as follows:
- From the model if already added by using Model.
- From the HTTP session by using
@SessionAttributes
.
- From a URI path variable passed through a Converter (see the next
example).
- From the invocation of a default constructor.
- From the invocation of a “primary constructor” with arguments that
match to Servlet request parameters. Argument names are determined
through JavaBeans@ConstructorProperties
or through runtime-retained
parameter names in the bytecode.
At this point this is the expected behavior and there might be good reasons or limitations behind that choice. Feel free to open an enhancement request in Spring Framework for that.
Thanks Brian, I'll do that !
– Julien O
Nov 13 at 16:25
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
The Spring WebFlux reference documentation about @ModelAttribute
does not mention URI path variables, unlike the same section in the reference documentation for Spring MVC:
The Pet instance above is resolved as follows:
- From the model if already added by using Model.
- From the HTTP session by using
@SessionAttributes
.
- From a URI path variable passed through a Converter (see the next
example).
- From the invocation of a default constructor.
- From the invocation of a “primary constructor” with arguments that
match to Servlet request parameters. Argument names are determined
through JavaBeans@ConstructorProperties
or through runtime-retained
parameter names in the bytecode.
At this point this is the expected behavior and there might be good reasons or limitations behind that choice. Feel free to open an enhancement request in Spring Framework for that.
Thanks Brian, I'll do that !
– Julien O
Nov 13 at 16:25
add a comment |
up vote
1
down vote
The Spring WebFlux reference documentation about @ModelAttribute
does not mention URI path variables, unlike the same section in the reference documentation for Spring MVC:
The Pet instance above is resolved as follows:
- From the model if already added by using Model.
- From the HTTP session by using
@SessionAttributes
.
- From a URI path variable passed through a Converter (see the next
example).
- From the invocation of a default constructor.
- From the invocation of a “primary constructor” with arguments that
match to Servlet request parameters. Argument names are determined
through JavaBeans@ConstructorProperties
or through runtime-retained
parameter names in the bytecode.
At this point this is the expected behavior and there might be good reasons or limitations behind that choice. Feel free to open an enhancement request in Spring Framework for that.
Thanks Brian, I'll do that !
– Julien O
Nov 13 at 16:25
add a comment |
up vote
1
down vote
up vote
1
down vote
The Spring WebFlux reference documentation about @ModelAttribute
does not mention URI path variables, unlike the same section in the reference documentation for Spring MVC:
The Pet instance above is resolved as follows:
- From the model if already added by using Model.
- From the HTTP session by using
@SessionAttributes
.
- From a URI path variable passed through a Converter (see the next
example).
- From the invocation of a default constructor.
- From the invocation of a “primary constructor” with arguments that
match to Servlet request parameters. Argument names are determined
through JavaBeans@ConstructorProperties
or through runtime-retained
parameter names in the bytecode.
At this point this is the expected behavior and there might be good reasons or limitations behind that choice. Feel free to open an enhancement request in Spring Framework for that.
The Spring WebFlux reference documentation about @ModelAttribute
does not mention URI path variables, unlike the same section in the reference documentation for Spring MVC:
The Pet instance above is resolved as follows:
- From the model if already added by using Model.
- From the HTTP session by using
@SessionAttributes
.
- From a URI path variable passed through a Converter (see the next
example).
- From the invocation of a default constructor.
- From the invocation of a “primary constructor” with arguments that
match to Servlet request parameters. Argument names are determined
through JavaBeans@ConstructorProperties
or through runtime-retained
parameter names in the bytecode.
At this point this is the expected behavior and there might be good reasons or limitations behind that choice. Feel free to open an enhancement request in Spring Framework for that.
answered Nov 12 at 8:21
Brian Clozel
29.3k67098
29.3k67098
Thanks Brian, I'll do that !
– Julien O
Nov 13 at 16:25
add a comment |
Thanks Brian, I'll do that !
– Julien O
Nov 13 at 16:25
Thanks Brian, I'll do that !
– Julien O
Nov 13 at 16:25
Thanks Brian, I'll do that !
– Julien O
Nov 13 at 16:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53253955%2fspring-webflux-doesnt-populate-custom-object-from-path-variables-with-modelatt%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fi z AlA 2Z Ejkd4xq 7PovWjDFu7zyb AoHPTK XDTnE,nwxPAmzgkK53ZhtPeeQ5Jt,n2Uv78Rtn3 Q2UD,VDgtBi