MVC - Multiple Parameter Ajax Call
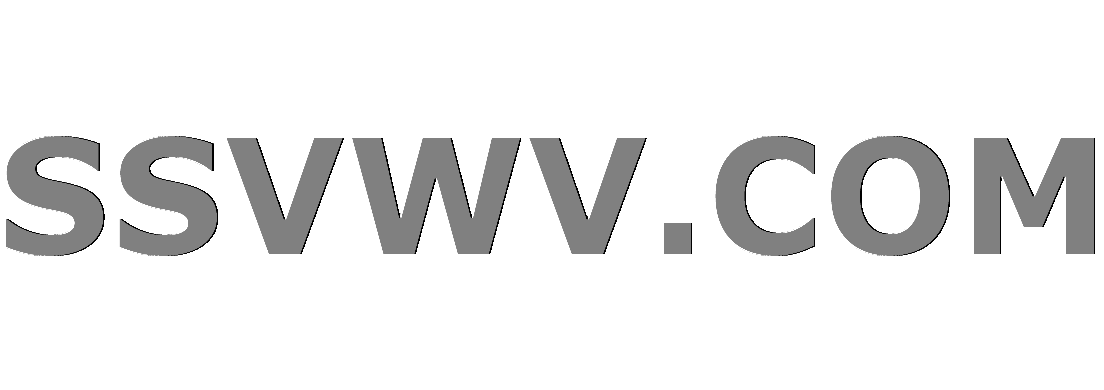
Multi tool use
I am trying to send 2 parameters to the controller from the View using an ajax call. This function worked earlier when I was only using 1 parameter but it no longer is working since I added a second.
Javascript with ajax:
function DeleteRole(roleId) {
var confirmation = confirm("Are you sure you want Delete this Role");
if (confirmation) {
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
roleId: roleId,
applicationId: $('#AppList').val()
})
}).success(function (response) {
if (response.success) {
alert(response.responseText);
$(".k-grid").data("kendoGrid").dataSource.read();
}else {
alert(response.responseText);
}
}).error(function() {
alert("Error on Deletion");
});
}
}
MVC - controller method(information isn't getting here at all)
[HttpPost]
public JsonResult Delete_Role(Guid rowId, Guid applicationId)
{
var users = new UserStore().ReadForAppRole(applicationId, rowId);
if (users.Any())
{
return Json(new { success = false, responseText = "Users's Currently Exist Within this Role" },
JsonRequestBehavior.AllowGet);
}
new RoleStore().RemoveRole(applicationId, rowId);
return Json(new { success = true, responseText = "Role Successfully Deleted" },
JsonRequestBehavior.AllowGet);
}
javascript ajax asp.net-mvc
add a comment |
I am trying to send 2 parameters to the controller from the View using an ajax call. This function worked earlier when I was only using 1 parameter but it no longer is working since I added a second.
Javascript with ajax:
function DeleteRole(roleId) {
var confirmation = confirm("Are you sure you want Delete this Role");
if (confirmation) {
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
roleId: roleId,
applicationId: $('#AppList').val()
})
}).success(function (response) {
if (response.success) {
alert(response.responseText);
$(".k-grid").data("kendoGrid").dataSource.read();
}else {
alert(response.responseText);
}
}).error(function() {
alert("Error on Deletion");
});
}
}
MVC - controller method(information isn't getting here at all)
[HttpPost]
public JsonResult Delete_Role(Guid rowId, Guid applicationId)
{
var users = new UserStore().ReadForAppRole(applicationId, rowId);
if (users.Any())
{
return Json(new { success = false, responseText = "Users's Currently Exist Within this Role" },
JsonRequestBehavior.AllowGet);
}
new RoleStore().RemoveRole(applicationId, rowId);
return Json(new { success = true, responseText = "Role Successfully Deleted" },
JsonRequestBehavior.AllowGet);
}
javascript ajax asp.net-mvc
2
you have arowId
vsroleId
the variable names need to match also not sure what happens if a non valid guid is passed - think it may just come in as null
– Pete
Nov 19 '18 at 16:17
Have you attempted to just change the end URL to a fake location or console logging the ajax request using a get global posts? And @Pete his remark fits too - the params could also be an issue but I personally would first attempt to find out if the post even occurs and then onward
– Le-Nerd tm
Nov 19 '18 at 16:18
1
you're sending a string of JSON but not telling the server that via the content-type. OTOH there's no particular need to send it as JSON, if you just remove the stringify operation then jQuery will form-encode it for you and MVC shouldn't have any trouble reading it.
– ADyson
Nov 19 '18 at 16:21
shouldn't delete be using[HttpDelete]
?
– Hanjun Chen
Nov 19 '18 at 16:26
add a comment |
I am trying to send 2 parameters to the controller from the View using an ajax call. This function worked earlier when I was only using 1 parameter but it no longer is working since I added a second.
Javascript with ajax:
function DeleteRole(roleId) {
var confirmation = confirm("Are you sure you want Delete this Role");
if (confirmation) {
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
roleId: roleId,
applicationId: $('#AppList').val()
})
}).success(function (response) {
if (response.success) {
alert(response.responseText);
$(".k-grid").data("kendoGrid").dataSource.read();
}else {
alert(response.responseText);
}
}).error(function() {
alert("Error on Deletion");
});
}
}
MVC - controller method(information isn't getting here at all)
[HttpPost]
public JsonResult Delete_Role(Guid rowId, Guid applicationId)
{
var users = new UserStore().ReadForAppRole(applicationId, rowId);
if (users.Any())
{
return Json(new { success = false, responseText = "Users's Currently Exist Within this Role" },
JsonRequestBehavior.AllowGet);
}
new RoleStore().RemoveRole(applicationId, rowId);
return Json(new { success = true, responseText = "Role Successfully Deleted" },
JsonRequestBehavior.AllowGet);
}
javascript ajax asp.net-mvc
I am trying to send 2 parameters to the controller from the View using an ajax call. This function worked earlier when I was only using 1 parameter but it no longer is working since I added a second.
Javascript with ajax:
function DeleteRole(roleId) {
var confirmation = confirm("Are you sure you want Delete this Role");
if (confirmation) {
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
roleId: roleId,
applicationId: $('#AppList').val()
})
}).success(function (response) {
if (response.success) {
alert(response.responseText);
$(".k-grid").data("kendoGrid").dataSource.read();
}else {
alert(response.responseText);
}
}).error(function() {
alert("Error on Deletion");
});
}
}
MVC - controller method(information isn't getting here at all)
[HttpPost]
public JsonResult Delete_Role(Guid rowId, Guid applicationId)
{
var users = new UserStore().ReadForAppRole(applicationId, rowId);
if (users.Any())
{
return Json(new { success = false, responseText = "Users's Currently Exist Within this Role" },
JsonRequestBehavior.AllowGet);
}
new RoleStore().RemoveRole(applicationId, rowId);
return Json(new { success = true, responseText = "Role Successfully Deleted" },
JsonRequestBehavior.AllowGet);
}
javascript ajax asp.net-mvc
javascript ajax asp.net-mvc
asked Nov 19 '18 at 16:12
crmckaincrmckain
786
786
2
you have arowId
vsroleId
the variable names need to match also not sure what happens if a non valid guid is passed - think it may just come in as null
– Pete
Nov 19 '18 at 16:17
Have you attempted to just change the end URL to a fake location or console logging the ajax request using a get global posts? And @Pete his remark fits too - the params could also be an issue but I personally would first attempt to find out if the post even occurs and then onward
– Le-Nerd tm
Nov 19 '18 at 16:18
1
you're sending a string of JSON but not telling the server that via the content-type. OTOH there's no particular need to send it as JSON, if you just remove the stringify operation then jQuery will form-encode it for you and MVC shouldn't have any trouble reading it.
– ADyson
Nov 19 '18 at 16:21
shouldn't delete be using[HttpDelete]
?
– Hanjun Chen
Nov 19 '18 at 16:26
add a comment |
2
you have arowId
vsroleId
the variable names need to match also not sure what happens if a non valid guid is passed - think it may just come in as null
– Pete
Nov 19 '18 at 16:17
Have you attempted to just change the end URL to a fake location or console logging the ajax request using a get global posts? And @Pete his remark fits too - the params could also be an issue but I personally would first attempt to find out if the post even occurs and then onward
– Le-Nerd tm
Nov 19 '18 at 16:18
1
you're sending a string of JSON but not telling the server that via the content-type. OTOH there's no particular need to send it as JSON, if you just remove the stringify operation then jQuery will form-encode it for you and MVC shouldn't have any trouble reading it.
– ADyson
Nov 19 '18 at 16:21
shouldn't delete be using[HttpDelete]
?
– Hanjun Chen
Nov 19 '18 at 16:26
2
2
you have a
rowId
vs roleId
the variable names need to match also not sure what happens if a non valid guid is passed - think it may just come in as null– Pete
Nov 19 '18 at 16:17
you have a
rowId
vs roleId
the variable names need to match also not sure what happens if a non valid guid is passed - think it may just come in as null– Pete
Nov 19 '18 at 16:17
Have you attempted to just change the end URL to a fake location or console logging the ajax request using a get global posts? And @Pete his remark fits too - the params could also be an issue but I personally would first attempt to find out if the post even occurs and then onward
– Le-Nerd tm
Nov 19 '18 at 16:18
Have you attempted to just change the end URL to a fake location or console logging the ajax request using a get global posts? And @Pete his remark fits too - the params could also be an issue but I personally would first attempt to find out if the post even occurs and then onward
– Le-Nerd tm
Nov 19 '18 at 16:18
1
1
you're sending a string of JSON but not telling the server that via the content-type. OTOH there's no particular need to send it as JSON, if you just remove the stringify operation then jQuery will form-encode it for you and MVC shouldn't have any trouble reading it.
– ADyson
Nov 19 '18 at 16:21
you're sending a string of JSON but not telling the server that via the content-type. OTOH there's no particular need to send it as JSON, if you just remove the stringify operation then jQuery will form-encode it for you and MVC shouldn't have any trouble reading it.
– ADyson
Nov 19 '18 at 16:21
shouldn't delete be using
[HttpDelete]
?– Hanjun Chen
Nov 19 '18 at 16:26
shouldn't delete be using
[HttpDelete]
?– Hanjun Chen
Nov 19 '18 at 16:26
add a comment |
1 Answer
1
active
oldest
votes
Two issues
1) Your action method parameter name is rowId
, but you are sending roleId
2) The JSON.stringify
method creates a string representation of the JavaScript object you pass to it. With this method, you are sending a JSON string of the object as the data
property of the $.ajax
method option. When sending a JSON string of your JS object, you should specify the contentType
property of the option to application/json
.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}),
contentType:"application/json"
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
Now $.ajax
will add the request header Content-Type
to the call with the value application/json
. As part of model binding, the default model binder will read this request header value and then decide to read the data from the request body(Request payload).
Also since you are not sending a complex object, you do not need to send the JSON string version. Simply pass the JavaScript object as the data
property and $.ajax
will send this as form data.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
data: {
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
In this case, $.ajax
will send application/x-www-form-urlencoded
as the Content-Type
request header value and model binder will be able to read it properly and map it your parameters.
You can also remove the dataType
in ajax call ( which I did in the second code snippet). jQuery ajax will guess the proper type from the response header and use that to further pars the data is received from the server call. In your case, you are calling the Json
method to return JSON response from your action method, which will send the application/json
as the Content-Type
header value.
thank you so much
– crmckain
Nov 19 '18 at 16:30
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378657%2fmvc-multiple-parameter-ajax-call%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Two issues
1) Your action method parameter name is rowId
, but you are sending roleId
2) The JSON.stringify
method creates a string representation of the JavaScript object you pass to it. With this method, you are sending a JSON string of the object as the data
property of the $.ajax
method option. When sending a JSON string of your JS object, you should specify the contentType
property of the option to application/json
.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}),
contentType:"application/json"
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
Now $.ajax
will add the request header Content-Type
to the call with the value application/json
. As part of model binding, the default model binder will read this request header value and then decide to read the data from the request body(Request payload).
Also since you are not sending a complex object, you do not need to send the JSON string version. Simply pass the JavaScript object as the data
property and $.ajax
will send this as form data.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
data: {
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
In this case, $.ajax
will send application/x-www-form-urlencoded
as the Content-Type
request header value and model binder will be able to read it properly and map it your parameters.
You can also remove the dataType
in ajax call ( which I did in the second code snippet). jQuery ajax will guess the proper type from the response header and use that to further pars the data is received from the server call. In your case, you are calling the Json
method to return JSON response from your action method, which will send the application/json
as the Content-Type
header value.
thank you so much
– crmckain
Nov 19 '18 at 16:30
add a comment |
Two issues
1) Your action method parameter name is rowId
, but you are sending roleId
2) The JSON.stringify
method creates a string representation of the JavaScript object you pass to it. With this method, you are sending a JSON string of the object as the data
property of the $.ajax
method option. When sending a JSON string of your JS object, you should specify the contentType
property of the option to application/json
.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}),
contentType:"application/json"
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
Now $.ajax
will add the request header Content-Type
to the call with the value application/json
. As part of model binding, the default model binder will read this request header value and then decide to read the data from the request body(Request payload).
Also since you are not sending a complex object, you do not need to send the JSON string version. Simply pass the JavaScript object as the data
property and $.ajax
will send this as form data.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
data: {
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
In this case, $.ajax
will send application/x-www-form-urlencoded
as the Content-Type
request header value and model binder will be able to read it properly and map it your parameters.
You can also remove the dataType
in ajax call ( which I did in the second code snippet). jQuery ajax will guess the proper type from the response header and use that to further pars the data is received from the server call. In your case, you are calling the Json
method to return JSON response from your action method, which will send the application/json
as the Content-Type
header value.
thank you so much
– crmckain
Nov 19 '18 at 16:30
add a comment |
Two issues
1) Your action method parameter name is rowId
, but you are sending roleId
2) The JSON.stringify
method creates a string representation of the JavaScript object you pass to it. With this method, you are sending a JSON string of the object as the data
property of the $.ajax
method option. When sending a JSON string of your JS object, you should specify the contentType
property of the option to application/json
.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}),
contentType:"application/json"
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
Now $.ajax
will add the request header Content-Type
to the call with the value application/json
. As part of model binding, the default model binder will read this request header value and then decide to read the data from the request body(Request payload).
Also since you are not sending a complex object, you do not need to send the JSON string version. Simply pass the JavaScript object as the data
property and $.ajax
will send this as form data.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
data: {
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
In this case, $.ajax
will send application/x-www-form-urlencoded
as the Content-Type
request header value and model binder will be able to read it properly and map it your parameters.
You can also remove the dataType
in ajax call ( which I did in the second code snippet). jQuery ajax will guess the proper type from the response header and use that to further pars the data is received from the server call. In your case, you are calling the Json
method to return JSON response from your action method, which will send the application/json
as the Content-Type
header value.
Two issues
1) Your action method parameter name is rowId
, but you are sending roleId
2) The JSON.stringify
method creates a string representation of the JavaScript object you pass to it. With this method, you are sending a JSON string of the object as the data
property of the $.ajax
method option. When sending a JSON string of your JS object, you should specify the contentType
property of the option to application/json
.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
dataType: 'json',
data: JSON.stringify({
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}),
contentType:"application/json"
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
Now $.ajax
will add the request header Content-Type
to the call with the value application/json
. As part of model binding, the default model binder will read this request header value and then decide to read the data from the request body(Request payload).
Also since you are not sending a complex object, you do not need to send the JSON string version. Simply pass the JavaScript object as the data
property and $.ajax
will send this as form data.
$.ajax({
type: "POST",
url: '@Url.Action("Delete_Role", "Admin")',
data: {
rowId: '@Guid.NewGuid()', // dummy GUID for testing
applicationId: '@Guid.NewGuid()'
}
}).done(function (response) {
console.log(response);
}).fail(function() {
console.log("Error on Deletion");
});
In this case, $.ajax
will send application/x-www-form-urlencoded
as the Content-Type
request header value and model binder will be able to read it properly and map it your parameters.
You can also remove the dataType
in ajax call ( which I did in the second code snippet). jQuery ajax will guess the proper type from the response header and use that to further pars the data is received from the server call. In your case, you are calling the Json
method to return JSON response from your action method, which will send the application/json
as the Content-Type
header value.
edited Nov 19 '18 at 17:47
answered Nov 19 '18 at 16:22
ShyjuShyju
145k87331437
145k87331437
thank you so much
– crmckain
Nov 19 '18 at 16:30
add a comment |
thank you so much
– crmckain
Nov 19 '18 at 16:30
thank you so much
– crmckain
Nov 19 '18 at 16:30
thank you so much
– crmckain
Nov 19 '18 at 16:30
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378657%2fmvc-multiple-parameter-ajax-call%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
crp8AhSHhWHOYm2NFv,lq,SAcIDRRwwqhcZ2DbCw1u,Rx55JfpppD,9Y
2
you have a
rowId
vsroleId
the variable names need to match also not sure what happens if a non valid guid is passed - think it may just come in as null– Pete
Nov 19 '18 at 16:17
Have you attempted to just change the end URL to a fake location or console logging the ajax request using a get global posts? And @Pete his remark fits too - the params could also be an issue but I personally would first attempt to find out if the post even occurs and then onward
– Le-Nerd tm
Nov 19 '18 at 16:18
1
you're sending a string of JSON but not telling the server that via the content-type. OTOH there's no particular need to send it as JSON, if you just remove the stringify operation then jQuery will form-encode it for you and MVC shouldn't have any trouble reading it.
– ADyson
Nov 19 '18 at 16:21
shouldn't delete be using
[HttpDelete]
?– Hanjun Chen
Nov 19 '18 at 16:26